Raspberry Pi Pico W LESSON 1: Write Your First Program for Absolute Beginners¶
Description¶
This video will walk you through the process of setting up MicroPython on your Raspberry Pi Pico W and installing Thonny, a user-friendly development environment, on your computer. With Thonny, you’ll be able to communicate directly with your Pico W. During this brief introductory session, you’ll create your first four programs and be given a homework task to complete. The class is perfect for total beginners—no prior experience is needed. My aim is to empower you with the skills to tackle these kinds of projects on your own, in a supportive and approachable way.
Hardware¶
# |
Component |
Description |
Tech Spec |
---|---|---|---|
1 |
Pi Pico W |
A microcontroller board with Wi-Fi capability, used to control the LED. |
3.3V operation, RP2040 CPU |
2 |
LED |
A light-emitting diode that blinks on and off as controlled by the Pico W. |
2V forward voltage, 20mA |
3 |
Capacitor |
Smooths out voltage fluctuations to stabilize the circuit. |
10µF, 16V (electrolytic) |
4 |
Breadboard |
A solder-less platform for building and testing the circuit layout. |
400 tie-points, 2.54mm pitch |
5 |
Wire |
Connects components on the breadboard to complete the circuit. |
22 AWG, solid core |
Check it out on WOKWI¶
MicroPython Simulation in Wokwi for VS Code¶
Based on Raspberry Pi Pico W Lessons for Absolute Beginners by Paul McWhorter¶
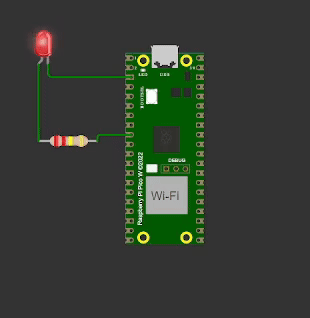
"""
Raspberry Pi Pico W LESSON 1: Write Your First Program for Absolute Beginners
https://www.youtube.com/watch?v=SL4_oU9t8Ss&list=PLGs0VKk2DiYz8js1SJog21cDhkBqyAhC5
"""
import time
from machine import Pin # pylint: disable=import-error
LED: Pin = Pin(6, Pin.OUT)
IS_ON: int = 0
def check_toggle_val(val: int) -> None:
"""
Valid val: 1, 0.
Raises exception in case of invalid val.
:param val:
:return:
"""
if not isinstance(val, int):
raise TypeError(f"Invalid parameter type: {type(val)}! Only integers allowed.")
if val not in (0, 1):
raise ValueError(f"Invalid parameter: {val}! Only 1 and 0 allowed.")
def toggle_is_on(val: int) -> int:
"""
Convert 1 to 0 and vice versa.
:param val:
:return:
"""
check_toggle_val(val)
return 1 if val == 0 else 0
def toggle_led(val: int) -> None:
"""
Toggle LED
:param val:
:return:
"""
check_toggle_val(val)
LED.value(val)
if __name__ == '__main__':
# Main loop
while True:
toggle_led(IS_ON)
time.sleep(0.3)
IS_ON = toggle_is_on(IS_ON)
{
"version": 1,
"author": "Egor Kostan",
"editor": "wokwi",
"parts": [
{
"type": "board-pi-pico-w",
"id": "pico",
"top": 0,
"left": 0,
"attrs": { "env": "micropython-20231227-v1.22.0" }
},
{
"type": "wokwi-led",
"id": "led1",
"top": -22.8,
"left": -101.8,
"attrs": { "color": "red", "flip": "1" }
},
{
"type": "wokwi-resistor",
"id": "r1",
"top": 90.35,
"left": -86.4,
"attrs": { "value": "220000" }
}
],
"connections": [
[ "led1:C", "pico:GND.1", "yellow", [ "v0" ] ],
[ "r1:1", "led1:A", "red", [ "v0" ] ],
[ "r1:2", "pico:GP6", "red", [ "v0" ] ]
],
"dependencies": {}
}